JavaScript vs TypeScript - Why We Use TypeScript
In 2022, TypeScript has rocketed up the charts and, according to the annual Stack Overflow survey, is sitting at #5 on the list of most popular scripting, programming and markup languages.
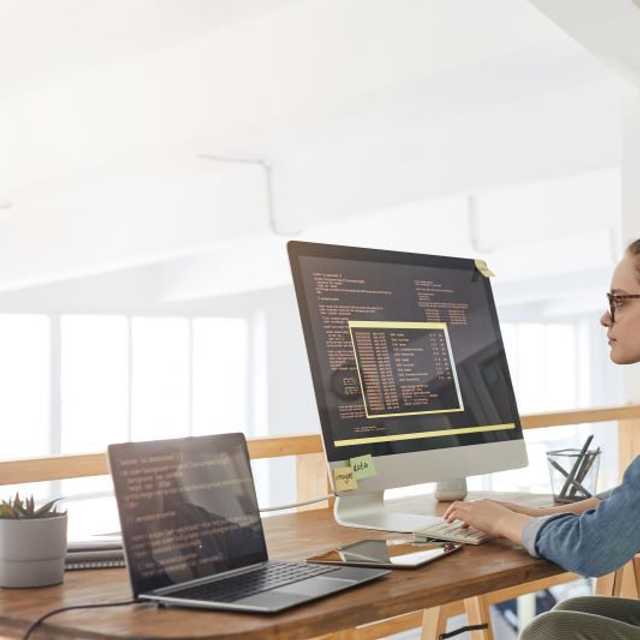
Typescript vs JavaScript
In 2022, TypeScript has rocketed up the charts and, according to the annual Stack Overflow survey, is sitting at #5 on the list of most popular scripting, programming and markup languages.
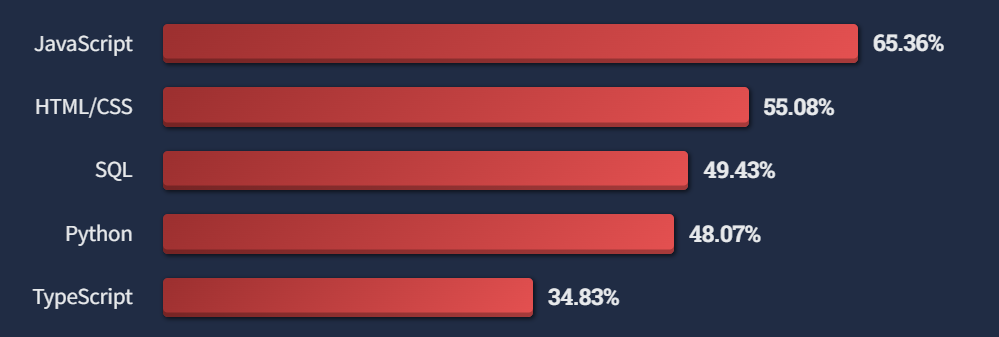
Tell me about TypeScript
Typescript is an object-orientated superset of JavaScript. In plain terms means TypeScript is JavaScript with some additional features baked in. It's compiled to JavaScript at runtime and is only an added development tool to make life easier for programmers.
Microsoft developed it back in 2013 to solve some of the problems of working with JavaScript.
TypeScript isn't trying to be a new, different version of JavaScript. It's really just an enhancement or an extension. Valid JavaScript code is valid TypeScript code.
What are the "problems" with writing JavaScript
JavaScript is a great language, but the way it handles data types is... well, kind of weird. JS was originally developed in just 10 days in 1995 and is backwards compatible. This means there are some syntax situations that can be real head-scratchers.
Here are a few examples.
Q: True + False
A: 1
Workings:
Number(true); // -> 1
Number(false); // -> 0
1 + 0; // -> 1
Q: (null - 0) + "0"
A: 00
Workings:
Number(null); // -> 0
0 - 0; // -> 0
0 + "0"; // -> "00"
Q: 1 + 2 + "3"
A: 33
Workings:
1 + 2; // -> 3
3 + "3"; // -> "33"
If you're unfamiliar with development, this might all seem like jibberish, but take a quick look at the last example. It's a simple maths scenario; 1 + 2 + 3. However, wrapping a number in inverted commas declares the value as a string (text), so JavaScript treats the equation as a string instead of a number.
The way JavaScript treats combined data types is not always clear, and as a result, it's not difficult to accidentally introduce time-consuming bugs.
IDE error catching isn't great
Integrated development environments (IDEs) are the applications where developers write their code. They provide tools that make the process easier, like nicely coloured classes, automatic indenting, live previews, and (argubaly) most importantly, error catching.
IDEs read code as it's written and underline problems as they're introduced. It's kind of like a spell-checker for code.
Error checkers for JavaScript have had to intentionally ignore problems related to type conflicts because they might not be a problem. The developer may have meant to combine a number and a string in the same function.
Bug fixing
Bug fixing large, complex projects written in JavaScript can be a nightmare because of the "grey area" I've hinted at above. Imagine looking through a 10,000-line project trying to spot a problem that's not actually being identified as an error.
As the size and complexity of a project increases, more rigid processes and checks need to be introduced to avoid chaos taking over.
How does TypeScript fix JavaScript?
TypeScript offers a way to define "types" as you're creating objects in JavaScript. Here's a quick example listed on the official TypeScript website.
The first step is to define the object `user`:
const user = {
name: "Hayes",
id: 0,
};
Once the object has been defined, each property can be bound to a type. This is done through an "interface":
interface User {
name: string;
id: number;
}
With the types of each object property now defined, the object can be associated with the interface and bound to the types by using the syntax :typeName after the variable declaration.
const user: User = {
name: "Hayes",
id: 0,
};
Now that the property types have been defined, if you accidentally invalidate the interface declaration, your IDE will throw up an error telling you something is wrong.
TypeScript goes a fair bit deeper than just defining property types. It can do cool things like composing new types by combining other simple types or declaring types based on an object's shape.
Frequently asked TypeScript questions
Here are the answers to a few questions you might have about TypeScript.
Is TypeScript better than JavaScript?
Yes, it is, but keep in mind that TypeScript is JavaScript. It's a superset that allows types to be defined to avoid the confusion and accidental bugs that happen in JS by allowing types to be combined.
As an agency that builds custom software that sometimes has large, complex interfaces, we would argue that TypeScript is better than JavaScript because it helps prevent type-based errors.
Does React use TypeScript?
React doesn't support TypeScript to the same extent Next.js does, but it does support JSX which is a feature of React.
const element = <h1>Hello, world!</h1>;
If you want full TypeScript support, you'll need to use a React framework like Next.js or create-react-app.
Is TypeScript Object Oriented?
Yes, TypeScript is an object-orientated language. Like JavaScript, TypeScript is actually prototype-based, which means that it defines behaviour through a constructor function instead of having true classes.
Got more questions?
If you've still got questions about TypeScript and its relationship to JavaScript, leave them in the comments section below and we'll get back to you.
Tim Davidson
Tim is the face of the company. When you want to kick off a new project, or an update on your existing project, Tim is your man!